When it comes to debugging an embedded application, establishing a functional debugging environment can be challenging. More often than not, JTAG tools are either proprietary and expensive, or free but difficult to use, and sometimes even ineffective. Ignoring the cost factor, professional JTAG software can be overly complex and cumbersome to automate.
These issues frequently lead developers from smaller companies or hobbyists to rely on a working console output and resort to basic ‘printf’ debugging. Scrutiny presents a viable alternative for efficient debugging and tuning of an embedded application.
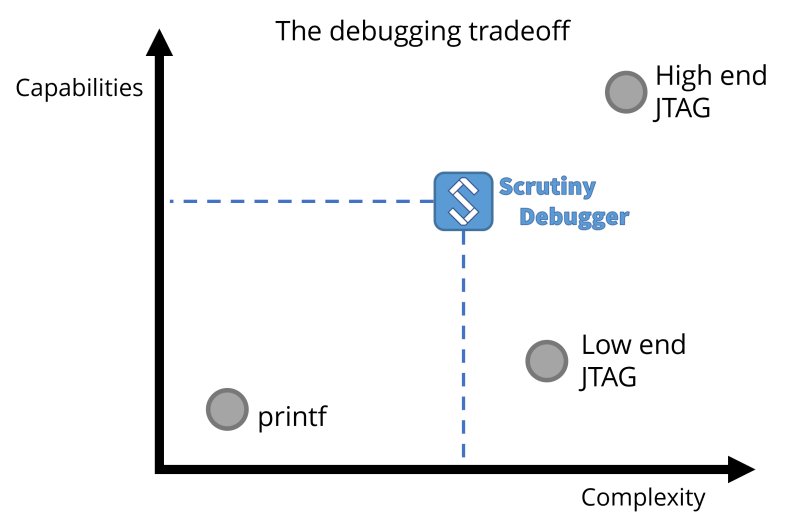
Certain applications are critical and cannot be halted or accessed via JTAG while in operation. A good example would be a drone controller. As the drone is in flight, the debugging process must not interfere with the application to prevent potential hardware damage. Obviously, connecting a wired JTAG is hardly feasible during flight.
So how do we handle this? Scrutiny does not interfere with the original application, because it works through instrumentation. Breakpoints are not possible, and memory accesses occur synchronously within the embedded application. The developer dictates when Scrutiny instrumentation's is executed, and sets its execution priority. Memory regions can be marked as forbidden or read-only, to avoid tampering with any critical memory section.
Lastly, Scrutiny operates through the communication channel already present on your hardware. Therefore, if you have Wifi, or a wireless serial port, Scrutiny can utilize that channel to communicate with its debug server. There's no need for additional proprietary hardware.